Have you noticed since installing the Yeoman generator for SharePoint, when you add a web part to a new or existing project and specify the “No JavaScript framework” option, you get a web part who’s render()
method’s indentation is all messed up like this?
// omitted for brevity...
export default class HelloWorldWebPart extends BaseClientSideWebPart <IHelloWorldWebPartProps> {
public render(): void {
this.domElement.innerHTML = `
<div class="${ styles.helloWorld }">
<div class="${ styles.container }">
<div class="${ styles.row }">
<div class="${ styles.column }">
<span class="${ styles.title }">Welcome to SharePoint!</span>
<p class="${ styles.subTitle }">Customize SharePoint experiences using Web Parts.</p>
<p class="${ styles.description }">${escape(this.properties.description)}</p>
<a href="https://aka.ms/spfx" class="${ styles.button }">
<span class="${ styles.label }">Learn more</span>
</a>
</div>
</div>
</div>
</div>`;
}
protected get dataVersion(): Version {
return Version.parse('1.0');
}
// omitted for brevity...
This is frustrating, but it’s very easy to fix.
The Yeoman generator for SharePoint is a globally installed npm package. When you execute yo @microsoft/sharepoint, after answering a few question prompts, the Yeoman uses template files to generate the new web part file.
It seems someone screwed up the indentation formatting & didn’t catch it before publishing the most recent generator so now, every time you create a new web part, this is what you get.
While Microsoft will likely fix this when they publish a new version of the generator until then you’re left with these ugly render()
methods.
Thankfully, it’s pretty easy to fix this… and in this post, I’ll show you how. It involves updating the templates in the generator.
In the future, when Microsoft publishes a new version of the SPFx generator, all your changes will be replaced with the new generator’s changes. This means you can think of this as a temporary change until Microsoft cleans up the default code created for a web part in a future version of the Yeoman generator for SharePoint.
This Process Is Non-Destructive - You Can Easily Revert Back
Don’t be scared to make this change to your development environment. You can easily undo this action if things don’t go well. The easiest way to undo these changes is to simply reinstall the generator by running npm install @microsoft/generator-sharepoint -g.
Locate the globally installed Yeoman generator for SharePoint
The first step is to locate the folder on your environment where the Yeoman generator for SharePoint is installed. All npm packages globally installed on your machine are placed in a centralized location.
An easy way to determine the location is to execute the npm help command: npm -h. At the bottom of the help, the last line of the output will contain a path to where npm is globally installed.
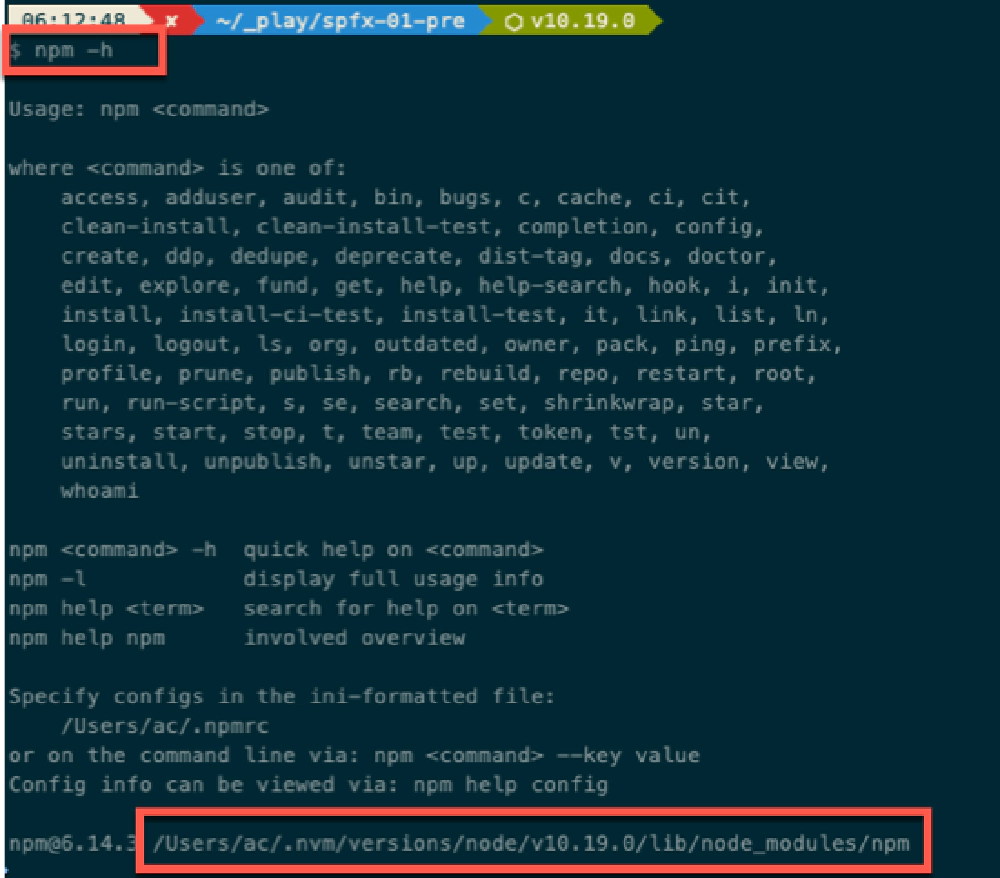
Output from running **npm -h** on MacOS with an NVM-managed Node.js v10.19.0 installation
On my laptop, npm is installed at the following location: /Users/ac/.nvm/versions/node/v10.19.0/lib/node_modules/npm. The location on your machine will be different based on two factors:
- Your operating system: Windows/MacOS/Linux
- If you installed Node.js with an installer or are using a tool like the Node Version Manager (NVM) like I am (read more why I love NVM)
Take the path reported by npm and remove npm from the end. The node_modules folder is where all packages are globally installed. The Yeoman generator for SharePoint is in the @microsoft/generator-sharepoint subfolder in there. So, on my laptop, I’m looking for this path: /Users/ac/.nvm/versions/node/v10.19.0/lib/node_modules/@microsoft/generator-sharepoint.
Update the Yeoman generator’s web part code template
Within the generator’s path, look for the following file: lib/generators/webpart/templates/none/{componentClassName}.ts. This is the file that’s used as the template when creating a new webpart with the “No JavaScript framework” option. Update the render()
method in that file to look like the following:
// omitted for brevity...
export default class <%= componentClassName %> extends BaseClientSideWebPart <I<%= componentClassName %>Props> {
public render(): void {
this.domElement.innerHTML = `
<div class="${ styles.<%= componentNameCamelCase %> }">
<div class="${ styles.container }">
<div class="${ styles.row }">
<div class="${ styles.column }">
<span class="${ styles.title }">Welcome to SharePoint!</span>
<p class="${ styles.subTitle }">Customize SharePoint experiences using Web Parts.</p>
<p class="${ styles.description }">${escape(this.properties.description)}</p>
<a href="https://aka.ms/spfx" class="${ styles.button }">
<span class="${ styles.label }">Learn more</span>
</a>
</div>
</div>
</div>
</div>`;
}
protected get dataVersion(): Version {
return Version.parse('1.0');
}
// omitted for brevity...
Save your changes to the file & test it. Simply create a new project using the generator and you’ll see the updated code template applied.

Microsoft MVP, Full-Stack Developer & Chief Course Artisan - Voitanos LLC.
Andrew Connell is a full stack developer who focuses on Microsoft Azure & Microsoft 365. He’s a 21-year recipient of Microsoft’s MVP award and has helped thousands of developers through the various courses he’s authored & taught. Whether it’s an introduction to the entire ecosystem, or a deep dive into a specific software, his resources, tools, and support help web developers become experts in the Microsoft 365 ecosystem, so they can become irreplaceable in their organization.