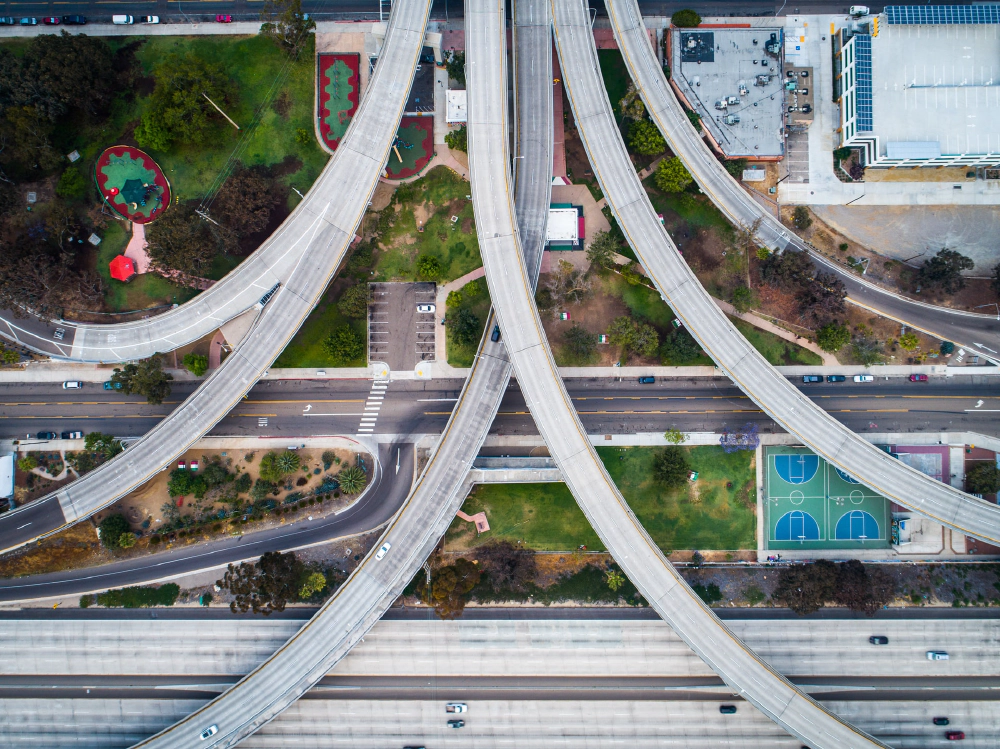
SharePoint Framework (SPFx) projects are written in TypeScript. For a while, developers were stuck using the version of TypeScript specified by Microsoft with each SPFx release. In the SPFx v1.8 release, the version of TypeScript was decoupled from the project so that now you can use any version you like, provided there’s a supported option. In this post, I’ll explain how this works, what versions of TypeScript you can use and how to implement it in your project.
The relationship between the SPFx development toolchain & TypeScript
Before I dive into this topic, I want to first explain why it’s not as simple as just updating the your project to a different TypeScript version. SPFx projects, or more correctly stated - the entire build toolchain, is built on multiple dependencies from multiple teams across Microsoft. These dependencies, things such as gulp tasks, are all written in TypeScript and used by multiple projects & teams, not just the SPFx team & projects.
When you build a SPFx project, there are multiple things built at the same time and these projects all need to be built in a specific order. Microsoft uses a home-grown tool, Rush, to build multiple projects in the desired order. Rush is similar to the popular tool Lerna that accomplishes much of the same thing.
So… SPFx projects, Rush, TypeScript… how do they all come together?
Rush Stack Compiler - decoupling the TypeScript compiler
For a while, up through the SPFx v1.7 release, the version of TypeScript was tied to the project. But in SPFx v1.8, the version of TypeScript was decoupled from the project by introducing a new npm package: The Rush Stack Compiler.
The Rush Stack Compiler isn’t just one package, but a series. There’s a different version available for each version of TypeScript. You can tell which version of TypeScript each version is tied to by the name of the package. For example, @microsoft/rush-stack-compiler-3.3 uses TypeScript v3.3.
SPFx & the Rush Stack Compiler
Each SPFx project can specify the version of TypeScript to use. In the current version of SPFx, version 1.11, the default project is configured to use TypeScript v3.3.
The TypeScript version must be set in two places:
The appropriate Rush Stack Compiler project must be installed and set as a dependency, ideally a
devDependency
, in the project.Take care to use the correct version that I specify below as some package versions don’t work with the SPFx project or it’s toolchain dependencies. In the code below, notice that while we’re using TypeScript 3.3 and the corresponding Rush Stack Compiler, notice we’re using version v0.3.5. There are multiple version of @microsoft/rush-stack-compiler-3.3 and not all work with the SPFx project or it’s toolchain, but v0.3.5 does work, so you want to be specific.
SPFx project’s package.json:
{ "devDependencies": { "@microsoft/rush-stack-compiler-3.3": "0.3.5", } }
The baseline tsconfig.json TypeScript compiler settings
The TypeScript compiler settings are set in one of two ways. Either you set them as arguments when you run the compiler, or you can set them in a project file. This project file, tscconfig.json is passed into the TypeScript compiler (TSC) when you run it.
All SPFx projects contain a tscconfig.json file at the root. This file takes a default project file and extends it with additional values for a SPFx project. The default that’s extended is coming from the Rush Stack Compiler that’s installed. Notice the path to the file contains the Rush Stack Compiler name, which includes the version of TypeScript in the name.
SPFx project’s tsconfig.json:
{ "extends": "./node_modules/@microsoft/rush-stack-compiler-3.3/includes/tsconfig-web.json", "compilerOptions": { .. } }
HOWTO: Change the TypeScript Version for your SPFx Projects
When you want to change your project to use a different version of TypeScript from what you get out of the box, you need to do three things:
Uninstall the existing Rush Stack Compiler from your project.
npm uninstall @microsof/rush-stack-compiler-3.3
Install the desired version of the Rush Stack Compiler for your project. Take care to use an exact version and don’t include any of the special characters in the version that npm adds, such as
~
or^
. These tell npm “you can install a more recent version if one has been released”. Do this by adding the –save-exact argument to the npm install command:npm install @microsoft/[email protected] --save-dev --save-exact
Manually update the tsconfig.json file in your project by changing the
extends
property to point to the path to the new Rush Stack Compiler package you installed:SPFx project’s tsconfig.json:
{ "extends": "./node_modules/@microsoft/rush-stack-compiler-3.9/includes/tsconfig-web.json", "compilerOptions": { .. } }
Whenever making changes to plumbing like this, I like to do a clean install of my dependencies. Do this by deleting the node_modules folder and delete the package-lock.json file before re-running npm install. I’ve noticed the above steps sometimes remove the TypeScript compiler from the dependencies & the installation of a new Rush Stack Compiler doesn’t add it back. However, simply reinstalling everything cleanly will fix that issue.
SPFx supported Rush Stack Compiler versions
The fun part of this process is knowing which version of TypeScript & the Rush Stack Compiler will work with SPFx projects! Here’s a list that’s based on what Microsoft has stated is supported in their release notes for SPFx v1.8 (up to TypeScript v3.3), as well as what the community has figured out since then.
TypeScript v2.7
@microsoft/rush-stack-compiler-2.7 v0.5.7
npm install @microsoft/[email protected] --save-dev --save-exact
TypeScript v2.9
@microsoft/rush-stack-compiler-2.9 v0.6.8
npm install @microsoft/[email protected] --save-dev --save-exact
TypeScript v3.0
@microsoft/rush-stack-compiler-3.0 v0.5.9
npm install @microsoft/[email protected] --save-dev --save-exact
TypeScript v3.3
@microsoft/rush-stack-compiler-3.3 v0.1.6
npm install @microsoft/[email protected] --save-dev --save-exact
TypeScript v3.4
@microsoft/rush-stack-compiler-3.4 v0.8.37
npm install @microsoft/[email protected] --save-dev --save-exact
TypeScript v3.5
@microsoft/rush-stack-compiler-3.5 v0.8.37
npm install @microsoft/[email protected] --save-dev --save-exact
TypeScript v3.6
@microsoft/rush-stack-compiler-3.6 v0.6.37
npm install @microsoft/[email protected] --save-dev --save-exact
TypeScript v3.7
@microsoft/rush-stack-compiler-3.7 v0.6.37
npm install @microsoft/[email protected] --save-dev --save-exact
TypeScript v3.8
@microsoft/rush-stack-compiler-3.8 v0.4.37
npm install @microsoft/[email protected] --save-dev --save-exact
TypeScript v3.9
@microsoft/rush-stack-compiler-3.9 v0.4.37
npm install @microsoft/[email protected] --save-dev --save-exact
TypeScript v4.5
@microsoft/rush-stack-compiler-4.5 v0.2.2
npm install @microsoft/[email protected] --save-dev --save-exact
When building your project, you may see this error:
Error - [tslint] no-use-before-declare is deprecated. Since TypeScript 2.9. Please use the built-in compiler checks instead.
As it states, the TSLint rule no-use-before-declare is deprecated and you shouldn’t use it any longer. To remove this error, locate this rule in the project’s ./tslint.json file and delete it.